SVGs (Scalable Vector Graphics) are a versatile format for creating and displaying vector-based graphics. Python makes it easy to manipulate SVG files dynamically for tasks like generating weekly menus. To deepen my knowledge about SVG manipulation in Python, I created a fictive menu template for a restaurant and wrote a script to insert the weekly menu together with the offering times.
Therefore I started with a very basic layout in Inkscape and wrote a script which replaces the text in the template by two weekly menus.
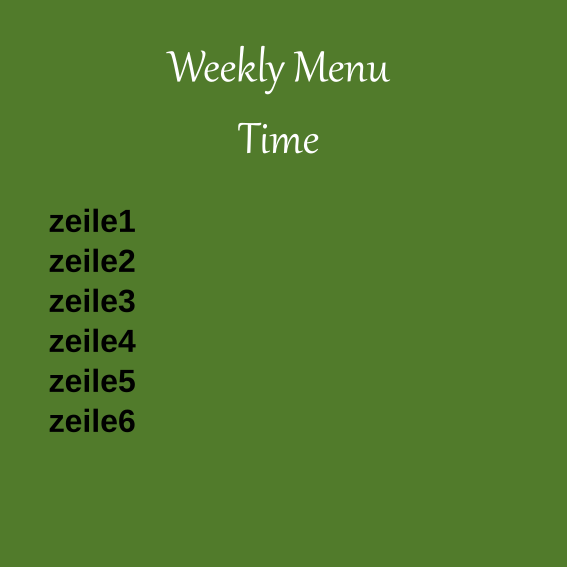
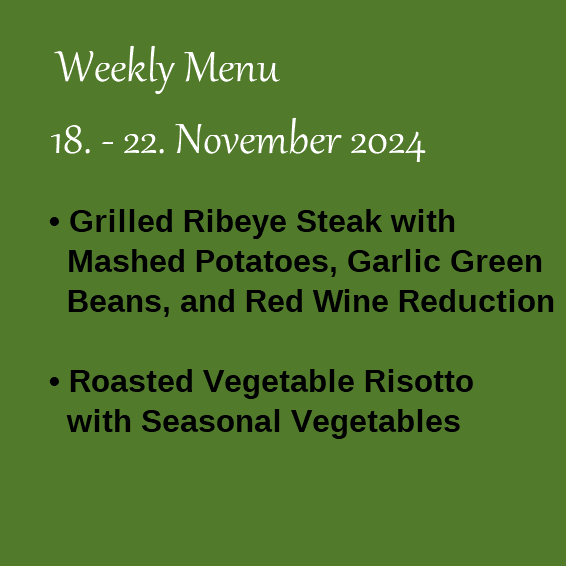
The script begins by defining the menu text and a time span. In a real-world scenario, user input (input()
) could be used for customization. For simplicity, uncommented hardcoded examples are provided as well.
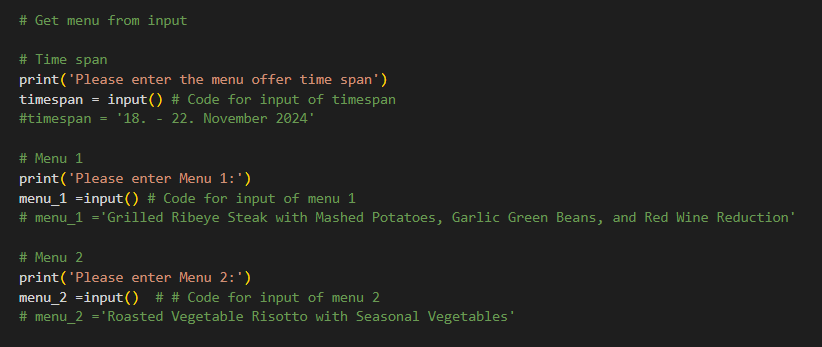
The most important code part was to replace the existing text by the new menu and writing the result into weekly_menu2.svg.
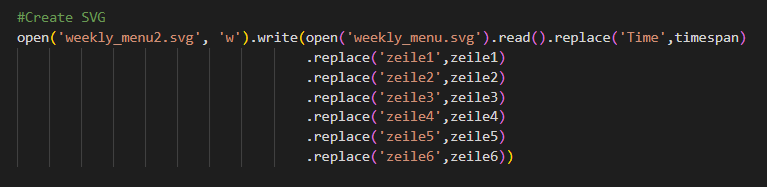
Therefore I replaced placeholders like Time
, zeile1
, etc. with the dynamically generated content.
During coding the script, I was also confronted with some hurdles, that I did not expect before stepping into the project:
- Unicode Character Conversion To ensure special characters (e.g., German umlauts) display correctly in SVG, the script converts them into their corresponding ASCII codes. This avoids rendering issues in non-standard fonts or software.
asci_dict = {'Ä': 196, 'ä': 228, 'Ö': 214, 'ö': 246, 'Ü': 220, 'ü': 252, 'ß': 223} for i in asci_dict.keys(): menu_1 = menu_1.replace(i, '&#' + str(asci_dict[i]) + ';') menu_2 = menu_2.replace(i, '&#' + str(asci_dict[i]) + ';')
- Dynamic Line Breaking Menus can vary in length, so the script dynamically inserts line breaks to ensure text fits neatly within the SVG design.
if len(menu_1) > 30: break_pos = menu_1.rfind(' ', 0, 30) zeile2 = menu_1[:break_pos] zeile3 = menu_1[break_pos:]
- SVG to PNG Conversion Using the
cairosvg
library, the code converts the final SVG file into a high-quality PNG image, ensuring compatibility with various platforms.svg2png(bytestring=open('weekly_menu2.svg').read(), write_to='weekly_menu2.png')
For further details about my svg text manipulation project, please refer to my GitHub repository.
In the next step, I want to automatically alter the names on my personalized flower pots as a preparation for offering my custom pots as stl-file on this website.